Aladdin SDK (SDK Integration)
It is recommended that the CODiScan SDK be used instead of SDK integration. The CODiScan SDK is more full featured and can run independently from the Aladdin app.
Overview
This section outlines the capabilities of our SDK, which facilitates seamless integration with the Android Aladdin application. The SDK provides a sophisticated subscription model, enabling developers to efficiently receive and manage scanned data. With built-in functionalities for both subscribe and unsubscribe operations, developers gain a professional and adaptable solution for incorporating scanned data into their applications.
Aladdin SDK
Integration
The Aladdin SDK is distributed through JitPack and can easily be referenced within a project's gradle build system.
Add jitpack.io repository
Add the jitpack repository to the repositories
block in the settings.gradle
file:
- Groovy
- Kotlin
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
mavenCentral()
maven(url="https://jitpack.io")
}
}
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
mavenCentral()
maven { url "https://jitpack.io" }
}
}
Add SDK dependency
Add a reference to the specific version of the SDK you would like to use to the build.gradle
file.
- Groovy
- Kotlin
dependencies {
...
implementation("com.github.datalogic:aladdin-app-sdk:{latest version}")
...
}
dependencies {
...
implementation 'com.github.datalogic:aladdin-app-sdk:{latest version}'
...
}
API
AlManager (Class)
Package: com.datalogic.aladdinsdk
Method | Summary |
---|---|
connectToService(context: Context): Boolean | Connect to the Aladdin App, if not connected already. Triggers a Service Binding via (AIDL) IPC to the Aladdin App. Will automatically re-establish the connection if necessary. |
disconnectFromService() | Disconnects the existing service binding. |
isConnectedToScanner(): Boolean | Returns if a scanner is currently connected to the Aladdin App or not. |
isConnectedToService(): Boolean | Returns if the Service is currently connected to the Aladdin App or not. |
subscribeToScans(callback: IScannerOutput) | Subscribe to scanner connection information and scanned barcodes. |
subscribeToServiceEvents(callback: IServiceOutput) | Subscribe to connection information of the sdk-service. |
unsubscribeFromScans(callback: IScannerOutput) | Unsubscribe from scanner connection information and scanned barcodes. |
unsubscribeFromServiceEvents(callback: IServiceOutput) | Unsubscribe connection information of the sdk-service. |
IScannerOutput (Interface)
Package: com.datalogic.aladdinsdk.interfaces
Method | Summary |
---|---|
onBarcodeScanned(data: BarcodeModel) | Callback Method that is called for each scanned barcode. It will only be called once per Barcode. |
onScannerConnected() | Callback method that is called once a scanner is connected to Aladdin App. |
onScannerDisconnected() | Callback method that is called once a scanner is disconnected from Aladdin App. |
IServiceOutput (Interface)
Package: com.datalogic.aladdinsdk.interfaces
Method | Summary |
---|---|
onServiceConnected() | Callback for the successful connection to the service. |
onServiceDisconnected() | Callback for a disconnection from the service. |
BarcodeModel (Class)
Package: com.datalogic.aladdinsdk.model
Method | Summary |
---|---|
getBarcode(): String | Returns the barcode data from the event object. |
getCode(): String | Returns the barcode type from the event object. |
getScanTime(): Long | Returns the time in milliseconds of the event object (based on the current device time). |
SDK Sample Client
The Aladdin SDK client is a purposefully crafted sample application designed to showcase the dynamic capabilities of the SDK. This app stands as a practical illustration of how the SDK integrates into Android environments. With an array of buttons representing distinct features, developers can interactively explore and understand the SDK's functionalities.
The client APK and source code can be found in the Aladdin samples repository on Github.
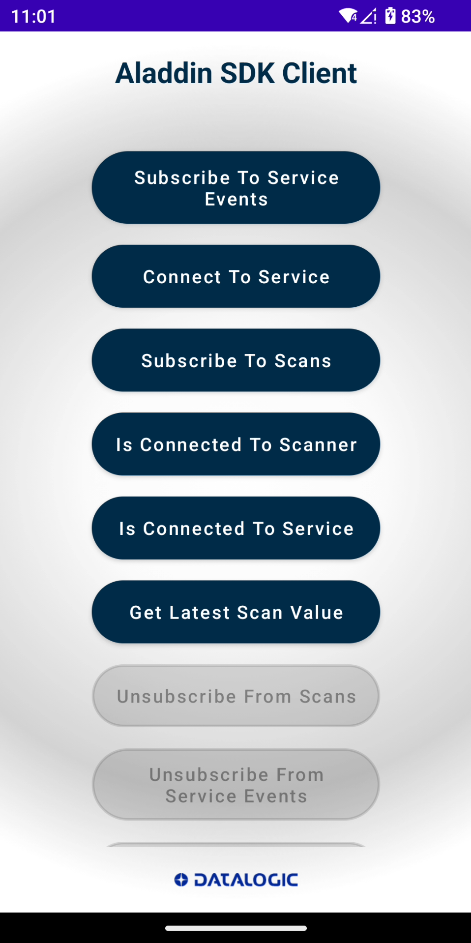
Exploring Button Capabilities
Subscribe To Service Events
Subscribe to connection information of the sdk-service. You will get notified when you are connected or disconnected from Aladdin application.
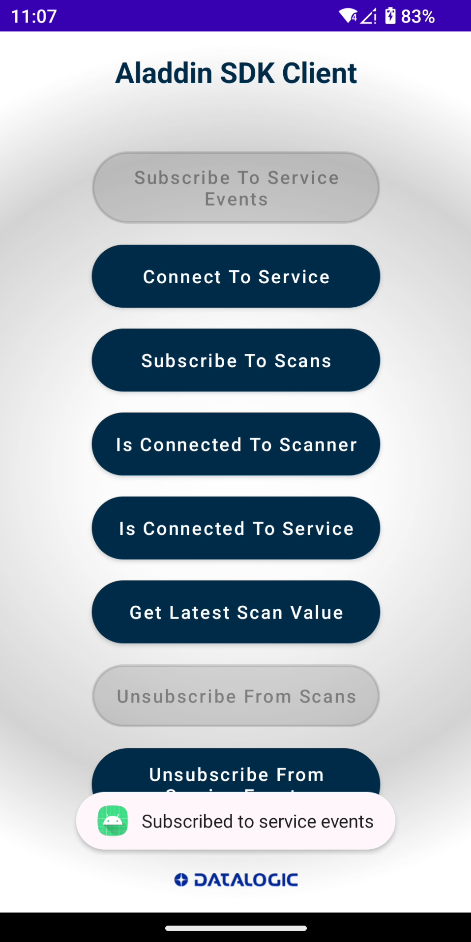
Connect To Service
Connect to the Aladdin application. Triggers a service binding via (AIDL) IPC to the Aladdin application.
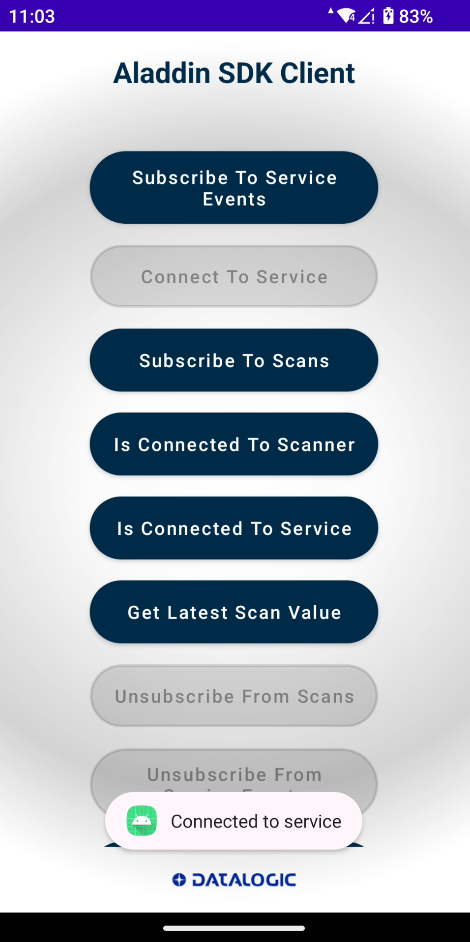
Subscribe To Scans
Subscribe to connection information and scanned barcodes. You will get notified when you are connected or disconnected from the CODiScan scanner and when a barcode is scanned (along with its data).
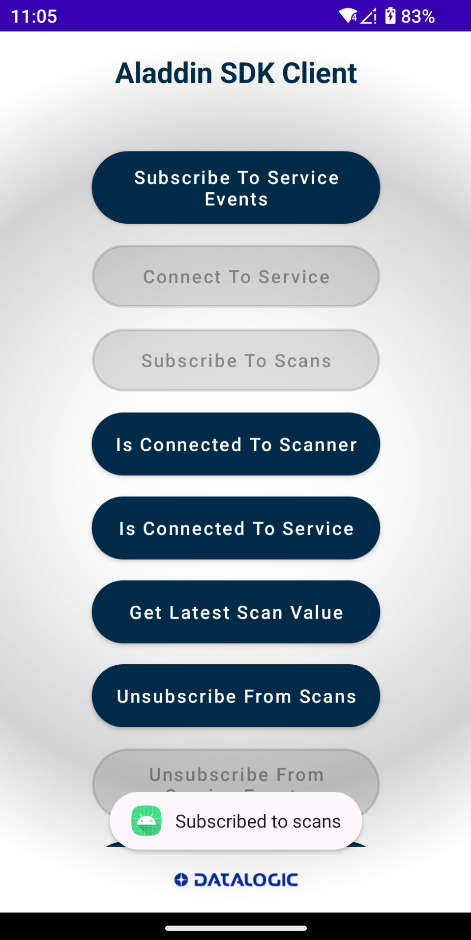
Is Connected To Scanner
Will get information related to scanner, whether it is currently connected to the Aladdin application or not.
Is Connected To Service
Will get information related to the service, whether it is currently connected to the Aladdin application or not.
Get Latest Scan Value
Get the last scanned value from the CODiScan.
Unsubscribe From Scans
Unsubscribe from connection information and scanned barcodes. You will not get any information related to scanned barcodes and CODiScan connections.
Unsubscribe From Service Events
Unsubscribe from connection information of the sdk-service. You will not get any information related to the connection with the Aladdin application.
Disconnect From Service
Disconnect from the Aladdin application. Removes the current service binding via (AIDL) IPC to the Aladdin application.
Steps to Receive Barcodes in Sample Client App
-
Install both applications (Aladdin and Sample Client) on the device.
-
Connect to Hand Scanner from Aladdin application and select "Integration method" as SDK.
-
Once the CODiScan is connected to the Aladdin application, open the Sample Client application.
-
Tap "Connect to service" in the client application. Once connection is successful, you will get connection toast message.
-
Tap "Subscribe to Scans" in client application.
-
Now you can scan the barcode data from the CODiScan device. You will get a toast message with scanned data in Sample Client application.